HTTP通信でリクエストを投げる
HTTP POST using Nefry BTこのページではNefry BTでHTTP POSTリクエストを扱うサンプルや記事を紹介します。
Nefry BTでシンプルなHTTP POSTリクエスト
HipChatのルームにPOSTするサンプルです。他のAPIでも応用できます。
curlでリクエスト送るとこんな感じです。
curl -d '{"color":"green","message":"test","notify":false,"message_format":"text"}' -H 'Content-Type: application/json' https://xxxxxx.hipchat.com/v2/room/4651875/notification?auth_token=xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
これをNefry BTで試してみましょう。 5秒に1回POSTするプログラムです。
#include <WiFiClientSecure.h>
WiFiClientSecure client;
const int HTTP_PORT = 443;
const char* HOST = "xxxxxx.hipchat.com";
void setup() {}
void loop() {
String url = "/v2/room/4651875/notification?auth_token=xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
url += "&color=green&message=test¬ify=false&message_format=text";
Serial.print("connecting to ");
Serial.println(HOST);
if (!client.connect(HOST, HTTP_PORT)) {
Serial.println("connection failed");
return;
}
client.println("POST " + url + " HTTP/1.1");
client.println("Content-Type: application/json");
client.println("Content-Type: application/x-www-form-urlencoded");
client.println("Host: " + String(HOST));
client.println("Connection: close");
client.println();
unsigned long timeout = millis();
while (client.available() == 0) {
if (millis() - timeout > 5000) {
Serial.println(">>> Client Timeout !");
client.stop();
return;
}
}
// Read all the lines of the reply from server and print them to Serial
while(client.available()) {
String line = client.readStringUntil('\r');
Serial.print(line);
}
Serial.println();
Serial.println("closing connection");
delay(5000);
}
実行結果はこんな感じです。
リクエストの要素にスペースや記号などが入る場合
例えば「My first notification (yey)」というメッセージを送りたい場合、スペースが入っていてURLのパラメータがうまく動いてくれない場合があります。
この場合はデフォルトプログラムのコードにもありますが、URLをエンコードする必要があります。
StrPerEncord
とescapeParameter
の関数でエンコードしています。
#include <WiFiClientSecure.h>
WiFiClientSecure client;
String StrPerEncord(const char* c_str);
String escapeParameter(String param);
const int HTTP_PORT = 443;
const char* HOST = "xxxxxx.hipchat.com";
void setup() {}
void loop() {
String url = "/v2/room/4651875/notification?auth_token=xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
url += "&color=green¬ify=false&message_format=text&message=";
url += StrPerEncord(escapeParameter("My first notification (yey)").c_str());
Serial.print("connecting to ");
Serial.println(HOST);
if (!client.connect(HOST, HTTP_PORT)) {
Serial.println("connection failed");
return;
}
client.println("POST " + url + " HTTP/1.1");
client.println("Content-Type: application/json");
client.println("Content-Type: application/x-www-form-urlencoded");
client.println("Host: " + String(HOST));
client.println("Connection: close");
client.println();
unsigned long timeout = millis();
while (client.available() == 0) {
if (millis() - timeout > 5000) {
Serial.println(">>> Client Timeout !");
client.stop();
return;
}
}
// Read all the lines of the reply from server and print them to Serial
while(client.available()) {
String line = client.readStringUntil('\r');
Serial.print(line);
}
Serial.println();
Serial.println("closing connection");
delay(5000);
}
String StrPerEncord(const char* c_str) {
uint16_t i = 0;
String str_ret = "";
char c1[3], c2[3], c3[3];
while (c_str[i] != '\0') {
if (c_str[i] >= 0xC2 && c_str[i] <= 0xD1) { //2バイト文字
sprintf(c1, "%2x", c_str[i]);
sprintf(c2, "%2x", c_str[i + 1]);
str_ret += "%" + String(c1) + "%" + String(c2);
i = i + 2;
} else if (c_str[i] >= 0xE2 && c_str[i] <= 0xEF) {
sprintf(c1, "%2x", c_str[i]);
sprintf(c2, "%2x", c_str[i + 1]);
sprintf(c3, "%2x", c_str[i + 2]);
str_ret += "%" + String(c1) + "%" + String(c2) + "%" + String(c3);
i = i + 3;
} else {
str_ret += String(c_str[i]);
i++;
}
}
return str_ret;
}
String escapeParameter(String param) {
param.replace("%", "%25");
param.replace("+", "%2B");
param.replace(" ", "+");
param.replace("\"", "%22");
param.replace("#", "%23");
param.replace("$", "%24");
param.replace("&", "%26");
param.replace("'", "%27");
param.replace("(", "%28");
param.replace(")", "%29");
param.replace("*", "%2A");
param.replace(",", "%2C");
param.replace("/", "%2F");
param.replace(":", "%3A");
param.replace(";", "%3B");
param.replace("<", "%3C");
param.replace("=", "%3D");
param.replace(">", "%3E");
param.replace("?", "%3F");
param.replace("@", "%40");
param.replace("[", "%5B");
param.replace("\\", "%5C");
param.replace("]", "%5D");
param.replace("^", "%5E");
param.replace("'", "%60");
param.replace("{", "%7B");
param.replace("|", "%7C");
param.replace("}", "%7D");
return param;
}
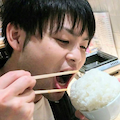